Imports and libraries
Using imports, it is possible to make libraries that can be used by multiple CIF specifications. For instance, consider the following CIF specification in file math.cif
:
// math.cif
func int inc(int x):
return x + 1;
end
This CIF specification declares a single function inc
that takes an integer number and returns that number incremented by one. Now also consider the following CIF specification in file counter.cif
:
// counter.cif
import "math.cif";
automaton counter:
disc int count = 0;
location:
initial;
edge tau do count := inc(count);
end
By importing the math.cif
file, the counter
automaton can use the inc
function. Other CIF files could similarly import the math.cif
file, essentially turning math.cif
into a library.
It is possible to make a function library, consisting of commonly used functions, a constant library, with commonly used constants, or an automaton definition library, with automaton definitions. As libraries are just CIF files, they can contain anything as long as they are valid CIF files. Essentially, every CIF file that is imported in more than one other CIF file can be considered a library.
The import as used above, only works if both CIF files are in the same directory. Library files however, are often placed in a different directory. Consider the same two CIF files, but organized into directories (or folders) as follows:
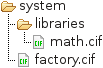
Directory system
contains a sub-directories named libraries
, which contains the math.cif
library. The system
directory also contains the counter.cif
file. The import in the counter.cif
file needs to be adapted to refer to the math.cif
file in the libraries
directory:
// counter.cif
import "libraries/math.cif";
automaton counter:
disc int count = 0;
location:
initial;
edge tau do count := inc(count);
end